스타일 서식 지정
import matplotlib.pyplot as plt
plt.style.use('default') # 기본 설정으로 초기화
print( plt.style.available ) # 스타일 서식 목록 출력
스타일 서식이 어떻게 적용되는지 알고 싶다면 여기
Style sheets reference — Matplotlib 3.5.1 documentation
Style sheets reference This script demonstrates the different available style sheets on a common set of example plots: scatter plot, image, bar graph, patches, line plot and histogram, import numpy as np import matplotlib.pyplot as plt # Fixing random stat
matplotlib.org
구성요소 다루기
Figure
plt.figure(figsize=(10,5), # 캔버스 크기 default : ( 6.4, 4.8 )
dpi=150, # Dots per inch 해상도 default : 100.0
facecolor='skyblue', # 면색 default : white
edgecolor='red', # 테두리색 default : white
linewidth=5 # 테두리두께 default : 0
)
print(plt.gcf()) # figure 객체 반환
plt.plot(range(1,5,1))
plt.show()
print(plt.gcf()) # figure 객체 반환
# plt객체는 show()를 하고 나면 다시 rcParams 값으로 초기화됨.
plt.figure()
plt.plot()
plt.show()
실행결과
Figure(1500x750)
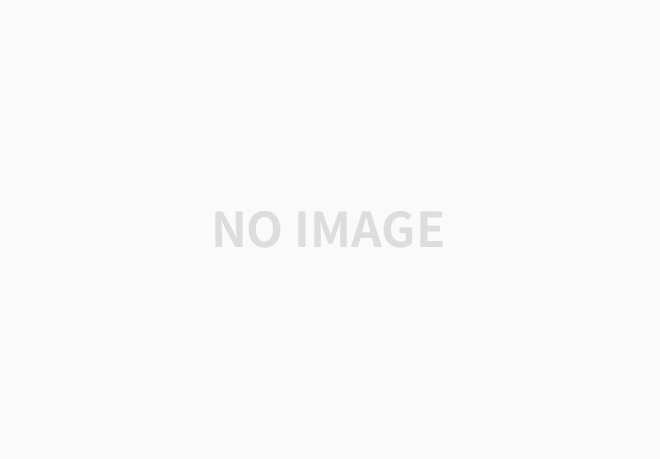
Figure(640x480)
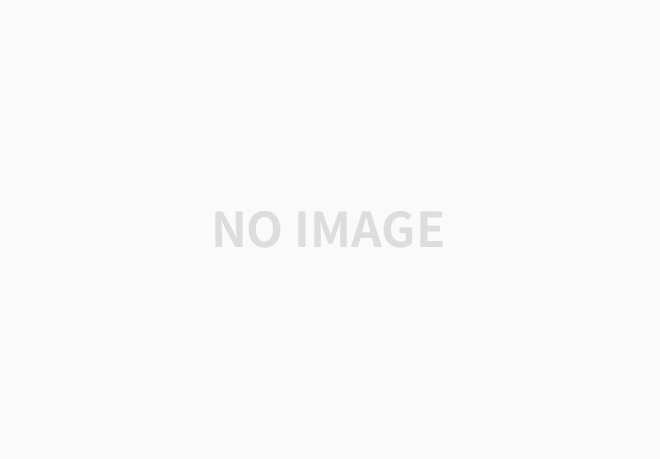
Figure 저장하고 불러오기
fig = plt.figure(figsize=(10,5), # 캔버스 크기 default : ( 6.4, 4.8 )
dpi=150, # Dots per inch 해상도 default : 100.0
facecolor='skyblue', # 면색 default : white
edgecolor='red', # 테두리색 default : white
linewidth=5 # 테두리두께 default : 0
)
print(fig)
plt.plot()
plt.show()
print(fig)
plt.figure(fig)
plt.show()
실행결과
Figure(1500x750)
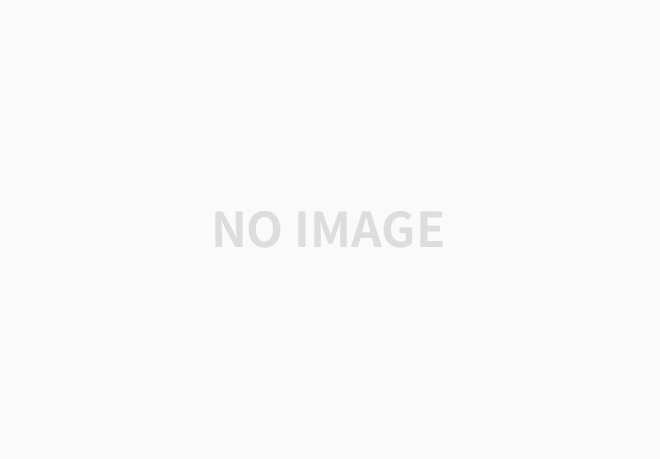
Figure(1500x750)
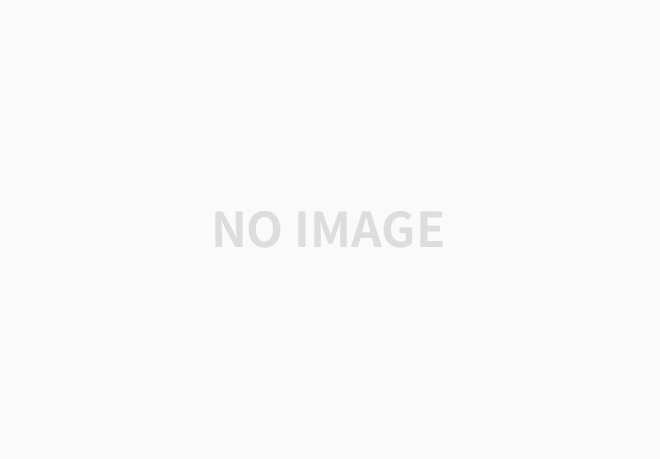
Axes ( **방법2** 로 하는 것을 추천 ; figure에 axes를 여러개 넣을 때 2번 방법이 유용함 )
Title 제목
title_font = {
'fontsize': 16, # 폰트 크기
'fontweight': 'bold' # 폰트 굵기
}
title(
'Sample graph', # 출력할 텍스트
fontdict=title_font, # 폰트 설정
loc='left', # 텍스트 위치
pad=20 # 텍스트와 Axes 사이 간격
)
# 방법1 matplotlib.pyplot
plt.title('Axes Title')
# 방법2 matplotlib.axes.Axes
ax = plt.gca() # matplotlib.axes.Axes 객체 반환
ax.set_title('Axes Title')
Legend 범례
legend(
*labels,
loc='best'
# 'best'(default), 'upper right', 'lower left', 'center', 'upper center', 'center left', ....
)
# 호출방법 4가지
# legend()
ax.legend()
# legend(handles, labels)
ax.legend([line1, line2, line3], ['label1', 'label2', 'label3'])
# legend(handles=handles)
line1, = ax.plot([1, 2, 3], label='label1')
line2, = ax.plot([1, 2, 3], label='label2')
ax.legend(handles=[line1, line2])
# legend(labels)
ax.plot([1, 2, 3])
ax.plot([5, 6, 7])
ax.legend(['First line', 'Second line'])
a = np.arange(0, 2, 0.2)
plt.plot(a, a, 'r--',
a, a**2, 'bo',
a, a**3, 'g-.')
plt.legend(["a", "a**2", "a**3"], loc='center')
plt.show()
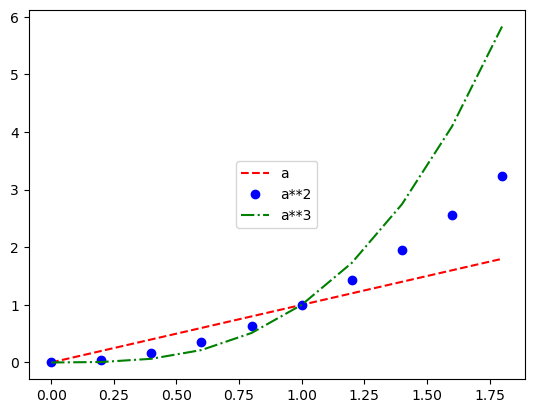
Axis label 축 라벨
# 방법1
plt.xlabel('x축') # x축이름 붙이기
plt.ylabel('y축') # y축이름 붙이기
# 방법2
ax = plt.gca()
ax.set_xlabel('x축')
ax.set_ylabel('y축')
Tick 눈금
tick_params(
axis='x', # 설정 축 선택 'both','x','y'
direction='in', # 눈금 방향 'out'(default), 'in', 'inout'
length=3, # 눈금 길이
pad=6, # 눈금과 눈금라벨 거리
labelsize=14, # 눈금라벨 크기
width=4, # 눈금폭
color='r', # 눈금색
labelcolor='green' # 눈금라벨색
)
ticks(
iterable, # 눈금을 표시할 값
iterable_label # 값에 표시할 라벨
)
# 방법1
plt.tick_params(axis='x', colors='blue') # axis = default : 'both' 가로세로축 동시설정
plt.tick_params(axis='y', colors='red')
plt.xticks([0,1,2]) # x축에 표시할 눈금
plt.yticks(arange(1,7,1)) # y축에 표시할 눈금
# 방법2
ax = plt.gca()
ax.tick_params(axis='x', colors='blue') # axis = default : 'both'
ax.tick_params(axis='y', colors='red')
ax.set_xticks([0,1,2]) # ax.set_xticklabels([0,1,2]) 와 같음
ax.set_yticks(arange(1,7,1)) # ax.set_yticklabels(arange(1,7,1)) 와 같음
a = np.arange(0, 2, 0.2)
plt.plot(a, a, 'bo')
plt.plot(a, a**2, color='#e35f62', marker='*', linewidth=2)
plt.plot(a, a**3, color='springgreen', marker='^', markersize=9)
plt.xticks(np.arange(0, 2.1, 0.2), labels=['Jan', '', 'Feb', '', 'Mar', '', 'May', '', 'June', '', 'July'])
plt.yticks(np.arange(0, 7), ('0', '1GB', '2GB', '3GB', '4GB', '5GB', '6GB'))
plt.tick_params(axis='x', direction='in', length=3, pad=6, labelsize=14, labelcolor='green')
plt.tick_params(axis='y', direction='inout', length=10, pad=15, labelsize=12, width=4, color='r')
plt.show()
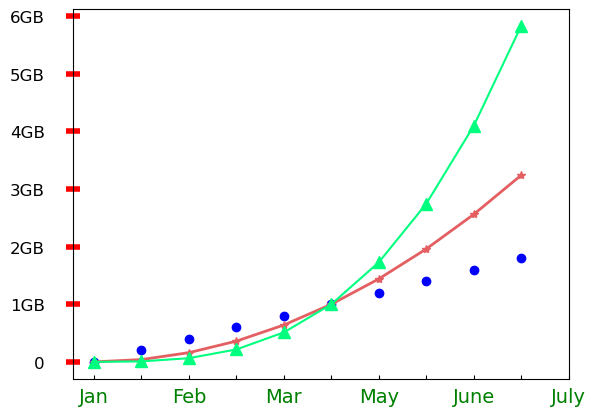
Gird 모눈(가로줄 세로줄)
grid(
# True, # 가시성 'None'(default), 'True', 'False' - 값에 상관없이 선 설정이 들어가면 선표시함.
# which='both' # 적용할 선 선택 'both', 'major', 'minor' - minor눈금을 쓰려면 로케이터에 대해서 알아야한다...
axis='y', # 축 'both', 'x', 'y'
color='red', # 선 색깔
alpha=0.5, # 선 투명도
linestyle='--' # 선 스타일
linewidth=1 # 선 두께
)
# 방법1
plt.grid()
# 방법2
ax = plt.gca()
ax.grid()
a = np.arange(0, 2, 0.2)
plt.plot(a, a, 'bo')
plt.grid(color='red', alpha=0.5, linestyle='--')
plt.show()

'Python 파이썬 > matplotlib' 카테고리의 다른 글
matplotlib ) 하나의 figure에 여러 개의 그래프 그리기 (0) | 2022.03.25 |
---|---|
matplotlib ) 라인 플롯(line plot) 스타일 지정 (0) | 2022.03.22 |
matplotlib ) 맷플롯립 개요 & 한글 폰트 오류 해결 (0) | 2022.03.21 |
댓글